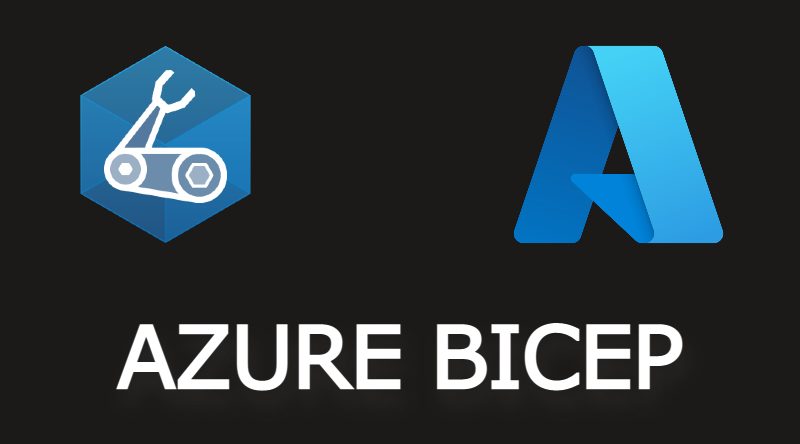
If you’re looking for a simple and efficient way to deploy your application on Azure, you’re in the right place. In this blog post, I will guide you through the process of deploying an existing docker image on Azure using Bicep. It’s easier than you might think, and a lot more fun too!
You can find all of the information discussed in this blog post in my GitHub repository, located at mhdbouk/libretranslate-bicep (github.com)
What is Bicep?
Bicep is a new language for deploying infrastructure on Azure. It is designed to be simple, clean, and easy to use, making it a powerful tool for developers/DevOps who want to deploy infrastructure on Azure quickly and efficiently.
The most powerful thing about bicep is its ability to automate the process of deploying infrastructure on Azure. With just a few lines of code, you can deploy complex infrastructure on Azure, saving you time and effort. Additionally, bicep allows you to manage and update your infrastructure in a simple and intuitive way, making it a valuable tool for any developer who works with Azure.
For this blog post, I’m going to deploy LibreTranslate, a free and open-source translation platform. LibreTranslate allows users to translate texts and documents into various languages. It uses machine learning algorithms to provide high-quality translations, and it is designed to be easy to use and customizable.
Ready, set, go
Before we dive into how to use Bicep, there are a few prerequisites you’ll need to have in place:
- An active Azure Subscription – you can sign up for free here
- Visual Studio Code
- Azure CLI & Bicep CLI
- OR Bicep VS Code extension
With these prerequisites in place, let’s get started
First, open VS Code and create a new file. We’ll call ours main.bicep
. Next, we’ll define the resources we need. In this example, we are going to create a new Azure Web App and a new Azure App Service Plan
resource appServicePlan 'Microsoft.Web/serverfarms@2020-12-01' = {
name: 'plan-demo-northeurope'
location: location
sku: {
name: 'F1'
capacity: 1
}
kind: 'linux'
properties: {
reserved: true
}
}
This resource means that we are going to create a new app service plan, Linux as an operating system, using the free plan.
We can make the values a parameter for the file by doing the following modification
@description('Describes plan\'s pricing tier and instance size. Check details at https://azure.microsoft.com/en-us/pricing/details/app-service/')
@allowed([
'F1'
'D1'
'B1'
'B2'
'B3'
'S1'
'S2'
'S3'
'P1'
'P2'
'P3'
'P4'
])
param skuName string = 'F1'
@description('Describes plan\'s instance count')
@minValue(1)
@maxValue(3)
param skuCapacity int = 1
@description('Location for all resources.')
param location string = resourceGroup().location
@description('App Service Plan Name.')
param appPlanName string = 'plan-demo-northeurope'
resource appServicePlan 'Microsoft.Web/serverfarms@2020-12-01' = {
name: appPlanName
location: location
sku: {
name: skuName
capacity: skuCapacity
}
kind: 'linux'
properties: {
reserved: true
}
}
Let us start deploying main.bicep
using Azure CLI and Bicep CLI
First, open your terminal and login into your Azure account using the Azure CLI
az login
Set the subscription that you want to use for the deployment (In case you have multiple associated to your account)
az account set --subscription YOUR_SUBSCRIPTION_ID
Use the Bicep CLI to build and deploy the main.bicep
file
bicep build main.bicep
az deployment group create --resource-group YOUR_RESOURCE_GROUP_NAME --template-file main.json
You should now have a successful deployment of main.bicep
on Azure. You can use the Azure portal or the Azure CLI to verify that the resources have been created as expected. You should see the new app service plan created.
To add an Azure Web App, add the following resource into main.bicep
resource webApplication 'Microsoft.Web/sites@2021-01-15' = {
name: appName
location: location
tags: {
'hidden-related:${resourceGroup().id}/providers/Microsoft.Web/serverfarms/appServicePlan': 'Resource'
}
properties: {
serverFarmId: appServicePlan.id
}
}
This modification will create the web app and link it to the app service plan. To configure app settings, the following can be used
resource webApplication 'Microsoft.Web/sites@2021-01-15' = {
name: appName
location: location
tags: {
'hidden-related:${resourceGroup().id}/providers/Microsoft.Web/serverfarms/appServicePlan': 'Resource'
}
properties: {
serverFarmId: appServicePlan.id
siteConfig: {
appSettings: [
{
name: 'My__Key'
value: 'My Value'
}
{
name: 'My__Other__Key'
value: otherKeyValue
}
]
}
}
}
And to add the docker image use the following
resource webApplication 'Microsoft.Web/sites@2021-01-15' = {
name: appName
location: location
tags: {
'hidden-related:${resourceGroup().id}/providers/Microsoft.Web/serverfarms/appServicePlan': 'Resource'
}
properties: {
serverFarmId: appServicePlan.id
siteConfig: {
linuxFxVersion: 'DOCKER|getting-started:latest'
appSettings: [
{
name: 'My__Key'
value: 'My Value'
}
{
name: 'My__Other__Key'
value: otherKeyValue
}
]
}
}
}
The following Gist contains all the needed resources in bicep to deploy LibraTranslate into Azure
When deploying this bicep
file to azure, it will create a new Linux-based Azure App Service Plan and configure a new Azure Web App with the necessary settings and configuration to run LibreTranslate from Docker
There is also an option to use your own Azure Container Registry (ACR), by changing the Container Registry
parameter to the URL of your custom registry.
Please note that in this example, LibreTranslate
will only be configured to start with support for English, Arabic, and Chinese languages.
In addition, I have included a Deploy To Azure
button in the GitHub repository: mhdbouk/libretranslate-bicep (github.com) to make it easy to deploy with just a single click